【C++庖丁解牛】C++11---lambda表达式 | 包装器
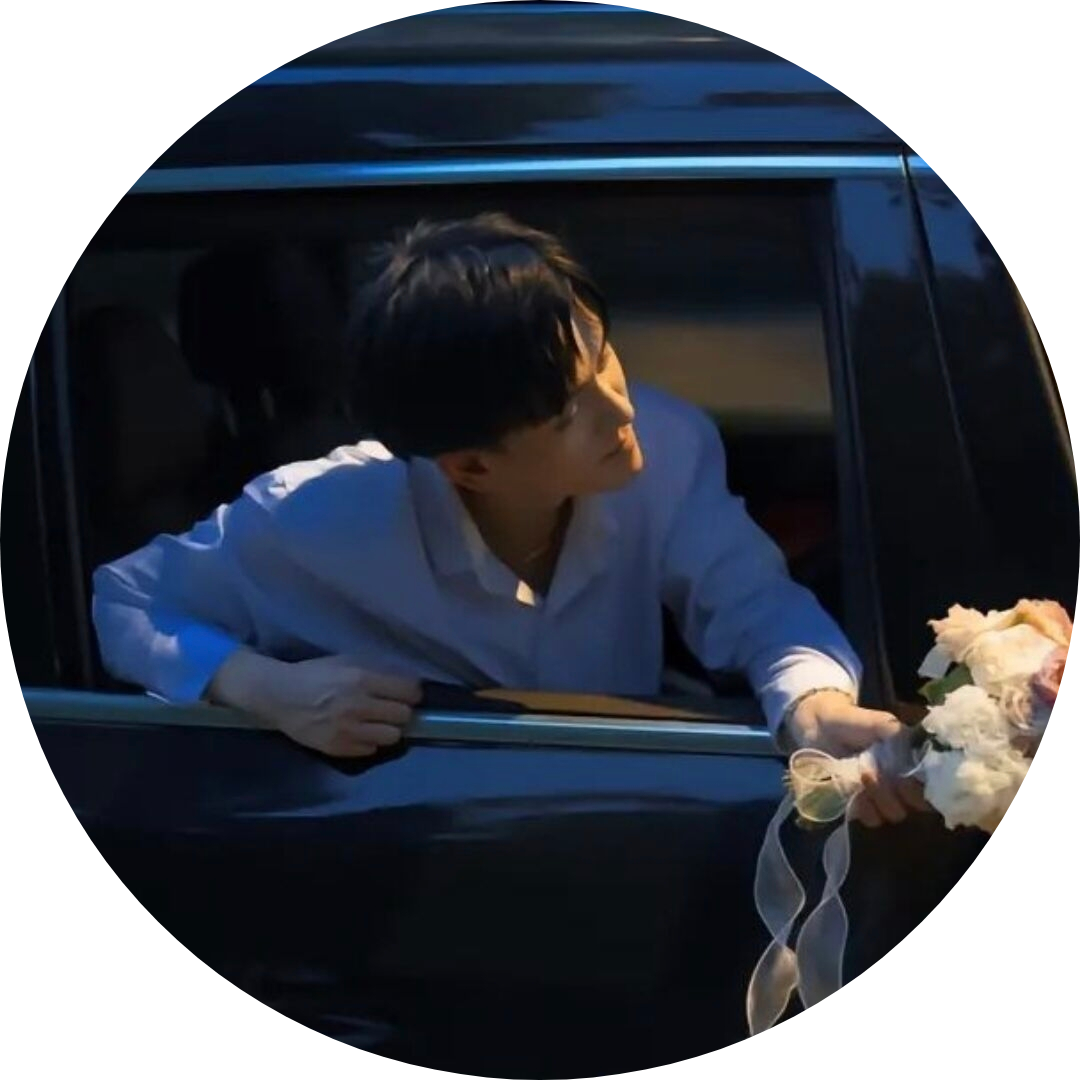
目录
- 1. lambda表达式
- 1.1 C++98中的一个例子
- 1.2 lambda表达式
- 1.3 lambda表达式语法
- 1.4. 捕获列表说明
- 1.5 函数对象与lambda表达式
- 2.包装器
- 2.1 bind
1. lambda表达式
1.1 C++98中的一个例子
在C++98中,如果想要对一个数据集合中的元素进行排序,可以使用std::sort方法。
#include #include int main() { int array[] = { 4,1,8,5,3,7,0,9,2,6 }; // 默认按照小于比较,排出来结果是升序 std::sort(array, array + sizeof(array) / sizeof(array[0])); // 如果需要降序,需要改变元素的比较规则 std::sort(array, array + sizeof(array) / sizeof(array[0]), greater()); return 0; }
在这里,greater是一个函数对象(function object),它是C++标准库中的一个模板类,用于比较两个元素的大小关系。greater是一个用于降序排序的比较器,它会将较大的元素排在前面。
如果待排序元素为自定义类型,需要用户定义排序时的比较规则:
#include using namespace std; #include #include #include #include struct Goods { string _name; // 名字 double _price; // 价格 int _evaluate; // 评价 Goods(const char* str, double price, int evaluate) :_name(str) , _price(price) , _evaluate(evaluate) {} }; struct ComparePriceLess { bool operator()(const Goods& gl, const Goods& gr) { return gl._price gr._price; } }; int main() { vector v = { { "苹果", 2.1, 5 }, { "香蕉", 3, 4 }, { "橙子", 2.2,3 }, { "菠萝", 1.5, 4 } }; sort(v.begin(), v.end(), ComparePriceLess()); sort(v.begin(), v.end(), ComparePriceGreater()); }
随着C++语法的发展,人们开始觉得上面的写法太复杂了,每次为了实现一个algorithm算法,都要重新去写一个类,如果每次比较的逻辑不一样,还要去实现多个类,特别是相同类的命名,这些都给编程者带来了极大的不便。因此,在C++11语法中出现了Lambda表达式。
1.2 lambda表达式
int main() { vector v = { { "苹果", 2.1, 5 }, { "香蕉", 3, 4 }, { "橙子", 2.2,3 }, { "菠萝", 1.5, 4 } }; sort(v.begin(), v.end(), [](const Goods& g1, const Goods& g2){ return g1._price g2._price; }); sort(v.begin(), v.end(), [](const Goods& g1, const Goods& g2) { return g1._evaluate g2._evaluate; }); }
上述代码就是使用C++11中的lambda表达式来解决,可以看出lambda表达式实际是一个匿名函数。
1.3 lambda表达式语法
lambda表达式书写格式:[capture-list] (parameters) mutable -> return-type { statement }
- lambda表达式各部分说明
- [capture-list] : 捕捉列表,该列表总是出现在lambda函数的开始位置,编译器根据[]来判断接下来的代码是否为lambda函数,捕捉列表能够捕捉上下文中的变量供lambda函数使用。
- (parameters):参数列表。与普通函数的参数列表一致,如果不需要参数传递,则可以连同()一起省略
- mutable:默认情况下,lambda函数总是一个const函数,mutable可以取消其常量性。使用该修饰符时,参数列表不可省略(即使参数为空)。
- ->returntype:返回值类型。用追踪返回类型形式声明函数的返回值类型,没有返回值时此部分可省略。返回值类型明确情况下,也可省略,由编译器对返回类型进行推导。
- {statement}:函数体。在该函数体内,除了可以使用其参数外,还可以使用所有捕获到的变量。
注意:
在lambda函数定义中,参数列表和返回值类型都是可选部分,而捕捉列表和函数体可以为空。因此C++11中最简单的lambda函数为:[]{}; 该lambda函数不能做任何事情。
int main() { //局部的匿名函数对象----简单的add函数 auto add = [](int a,int b)->int{return a+b}; cout}; // 省略参数列表和返回值类型,返回值类型由编译器推导为int int a = 3, b = 4; [=] {return a + 3; }; // 省略了返回值类型,无返回值类型 auto fun1 = [&](int c) {b = a + c; }; fun1(10); cout return b += a + c; }; //这里捕捉的是等于号也就是全局的变量,&b就是给b取别名便于给b进行修改操作 cout x *= 2; return a + x; }; cout auto f1 = [] {cout cout public: Rate(double rate) : _rate(rate) {} double operator()(double money, int year) { return money * _rate * year; } private: double _rate; }; int main() { // 函数对象 double rate = 0.49; Rate r1(rate); r1(10000, 2); // lamber auto r2 = [=](double monty, int year)-double {return monty * rate * year; }; r2(10000, 2); return 0; } static int count = 0; cout return i / 2; } struct Functor { double operator()(double d) { return d / 3; } }; int main() { // 函数名 cout return d / 4; }, 11.11) return a + b; } struct Functor { public: int operator() (int a, int b) { return a + b; } }; class Plus { public: static int plusi(int a, int b) { return a + b; } double plusd(double a, double b) { return a + b; } }; int main() { // 函数名(函数指针) std::functionreturn a + b; }; cout static int count = 0; cout return i / 2; } struct Functor { double operator()(double d) { return d / 3; } }; int main() { // 函数名 std::function return d /4; }; cout std::cout return a + b; } class Sub { public: int sub(int a, int b) { return a - b; } }; int main() { //表示绑定函数plus 参数分别由调用 func1 的第一,二个参数指定 std::function
- 2.1 bind
文章版权声明:除非注明,否则均为主机测评原创文章,转载或复制请以超链接形式并注明出处。